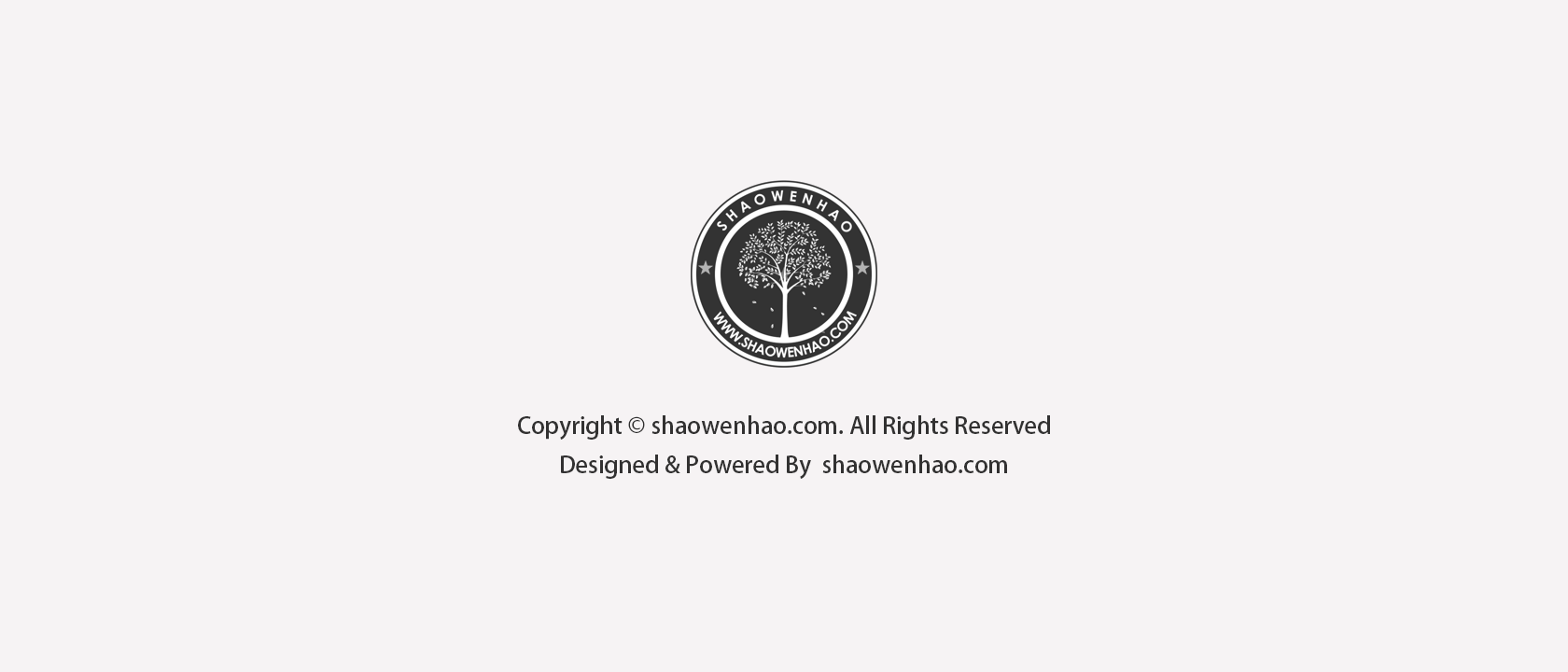
文章目录
什么是正则表达式?
正则表达式(Regular Expression)通常被用来检索、替换那些符合某个模式(规则)的文本。
此处的Regular即是规则、规律的意思,Regular Expression即“描述某种规则的表达式”之意。
本文收集了一些常见的正则表达式用法,方便大家查询取用,并在最后附了详细的正则表达式语法手册。
案例包括:邮箱、身份证号、手机号码、固定电话、域名、IP地址、日期、邮编、密码、中文字符、数字、字符串
Python如何支持正则?
我用的是python来实现正则,并使用Jupyter Notebook编写代码。
Python通过re模块支持正则表达式,re 模块使 Python 语言拥有全部的正则表达式功能。
这里要注意两个函数的使用:
re.compile
用于编译正则表达式,生成一个正则表达式( Pattern )对象;
.findall
用于在字符串中找到正则表达式所匹配的所有子串,并返回一个列表,如果没有找到匹配的,则返回空列表。
# 导入re模块
import re
1.邮箱
包含大小写字母,下划线,阿拉伯数字,点号,中划线
表达式:
[a-zA-Z0-9_-]+@[a-zA-Z0-9_-]+(?:\.[a-zA-Z0-9_-]+)
案例:
pattern = re.compile(r"[a-zA-Z0-9_-]+@[a-zA-Z0-9_-]+(?:\.[a-zA-Z0-9_-]+)")
strs = '我的私人邮箱是zhuwjwh@outlook.com,公司邮箱是123456@qq.org,麻烦登记一下?'
result = pattern.findall(strs)
print(result)
['zhuwjwh@outlook.com', '123456@qq.org']
2. 身份证号
xxxxxx yyyy MM dd 375 0 十八位
- 地区: [1-9]\d{5}
- 年的前两位: (18|19|([23]\d)) 1800-2399
- 年的后两位: \d{2}
- 月份: ((0[1-9])|(10|11|12))
- 天数: (([0-2][1-9])|10|20|30|31) 闰年不能禁止29+
- 三位顺序码: \d{3}
- 两位顺序码: \d{2}
- 校验码: [0-9Xx]
表达式:
[1-9]\d{5}(18|19|([23]\d))\d{2}((0[1-9])|(10|11|12))(([0-2][1-9])|10|20|30|31)\d{3}[0-9Xx]
案例:
pattern = re.compile(r"[1-9]\d{5}(?:18|19|(?:[23]\d))\d{2}(?:(?:0[1-9])|(?:10|11|12))(?:(?:[0-2][1-9])|10|20|30|31)\d{3}[0-9Xx]")
strs = '小明的身份证号码是342623198910235163,手机号是13987692110'
result = pattern.findall(strs)
print(result)
['342623198910235163']
3. 国内手机号码
手机号都为11位,且以1开头,第二位一般为3、5、6、7、8、9 ,剩下八位任意数字
例如:13987692110、15610098778
表达式:
1(3|4|5|6|7|8|9)\d{9}
案例:
pattern = re.compile(r"1[356789]\d{9}")
strs = '小明的手机号是13987692110,你明天打给他'
result = pattern.findall(strs)
print(result)
['13987692110']
4. 国内固定电话
区号3\~4位,号码7\~8位
例如:0511-1234567、021-87654321
表达式:
\d{3}-\d{8}|\d{4}-\d{7}
案例:
pattern = re.compile(r"\d{3}-\d{8}|\d{4}-\d{7}")
strs = '0511-1234567是小明家的电话,他的办公室电话是021-87654321'
result = pattern.findall(strs)
print(result)
['0511-1234567', '021-87654321']
5. 域名
包含http:\\或https:\\
表达式:
(?:(?:http:\/\/)|(?:https:\/\/))?(?:[\w](?:[\w\-]{0,61}[\w])?\.)+[a-zA-Z]{2,6}(?:\/)
案例:
pattern = re.compile(r"(?:(?:http:\/\/)|(?:https:\/\/))?(?:[\w](?:[\w\-]{0,61}[\w])?\.)+[a-zA-Z]{2,6}(?:\/)")
strs = 'Python官网的网址是https://www.python.org/'
result = pattern.findall(strs)
print(result)
['https://www.python.org/']
6. IP地址
IP地址的长度为32位(共有2^32个IP地址),分为4段,每段8位,用十进制数字表示
每段数字范围为0~255,段与段之间用句点隔开
表达式:
((?:(?:25[0-5]|2[0-4]\d|[01]?\d?\d)\.){3}(?:25[0-5]|2[0-4]\d|[01]?\d?\d))
案例:
pattern = re.compile(r"((?:(?:25[0-5]|2[0-4]\d|[01]?\d?\d)\.){3}(?:25[0-5]|2[0-4]\d|[01]?\d?\d))")
strs = '''请输入合法IP地址,非法IP地址和其他字符将被过滤!
增、删、改IP地址后,请保存、关闭记事本!
192.168.8.84
192.168.8.85
192.168.8.86
0.0.0.1
256.1.1.1
192.256.256.256
192.255.255.255
aa.bb.cc.dd'''
result = pattern.findall(strs)
print(result)
['192.168.8.84', '192.168.8.85', '192.168.8.86', '0.0.0.1', '56.1.1.1', '192.255.255.255']
7. 日期
常见日期格式:yyyyMMdd、yyyy-MM-dd、yyyy/MM/dd、yyyy.MM.dd
表达式:
\d{4}(?:-|\/|.)\d{1,2}(?:-|\/|.)\d{1,2}
案例:
pattern = re.compile(r"\d{4}(?:-|\/|.)\d{1,2}(?:-|\/|.)\d{1,2}")
strs = '今天是2020/12/20,去年的今天是2019.12.20,明年的今天是2021-12-20'
result = pattern.findall(strs)
print(result)
['2020/12/20', '2019.12.20', '2021-12-20']
8. 国内邮政编码
我国的邮政编码采用四级六位数编码结构
前两位数字表示省(直辖市、自治区)
第三位数字表示邮区;第四位数字表示县(市)
最后两位数字表示投递局(所)
表达式:
[1-9]\d{5}(?!\d)
案例:
pattern = re.compile(r"[1-9]\d{5}(?!\d)")
strs = '上海静安区邮编是200040'
result = pattern.findall(strs)
print(result)
['200040']
9. 密码
密码(以字母开头,长度在6~18之间,只能包含字母、数字和下划线)
表达式:
[a-zA-Z]\w{5,17}
强密码(以字母开头,必须包含大小写字母和数字的组合,不能使用特殊字符,长度在8-10之间)
表达式:
[a-zA-Z](?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{8,10}
pattern = re.compile(r"[a-zA-Z]\w{5,17}")
strs = '密码:q123456_abc'
result = pattern.findall(strs)
print(result)
['q123456_abc']
pattern = re.compile(r"[a-zA-Z](?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{8,10}")
strs = '强密码:q123456ABc,弱密码:q123456abc'
result = pattern.findall(strs)
print(result)
['q123456ABc,']
10. 中文字符
表达式:
[\u4e00-\u9fa5]
案例:
pattern = re.compile(r"[\u4e00-\u9fa5]")
strs = 'apple:苹果'
result = pattern.findall(strs)
print(result)
['苹', '果']
11. 数字
- 验证数字:
^[0-9]*$
- 验证n位的数字:
^\d{n}$
- 验证至少n位数字:
^\d{n,}$
- 验证m-n位的数字:
^\d{m,n}$
- 验证零和非零开头的数字:
^(0|[1-9][0-9]*)$
- 验证有两位小数的正实数:
^[0-9]+(.[0-9]{2})?$
- 验证有1-3位小数的正实数:
^[0-9]+(.[0-9]{1,3})?$
- 验证非零的正整数:
^\+?[1-9][0-9]*$
- 验证非零的负整数:
^\-[1-9][0-9]*$
- 验证非负整数(正整数 + 0)
^\d+$
- 验证非正整数(负整数 + 0)
^((-\d+)|(0+))$
- 整数:
^-?\d+$
- 非负浮点数(正浮点数 + 0):
^\d+(\.\d+)?$
- 正浮点数
^(([0-9]+\.[0-9]*[1-9][0-9]*)|([0-9]*[1-9][0-9]*\.[0-9]+)|([0-9]*[1-9][0-9]*))$
- 非正浮点数(负浮点数 + 0)
^((-\d+(\.\d+)?)|(0+(\.0+)?))$
- 负浮点数
^(-(([0-9]+\.[0-9]*[1-9][0-9]*)|([0-9]*[1-9][0-9]*\.[0-9]+)|([0-9]*[1-9][0-9]*)))$
- 浮点数
^(-?\d+)(\.\d+)?$
12. 字符串
- 英文和数字:
^[A-Za-z0-9]+$ 或 ^[A-Za-z0-9]{4,40}$
- 长度为3-20的所有字符:
^.{3,20}$
- 由26个英文字母组成的字符串:
^[A-Za-z]+$
- 由26个大写英文字母组成的字符串:
^[A-Z]+$
- 由26个小写英文字母组成的字符串:
^[a-z]+$
- 由数字和26个英文字母组成的字符串:
^[A-Za-z0-9]+$
- 由数字、26个英文字母或者下划线组成的字符串:
^\w+$ 或 ^\w{3,20}$
- 中文、英文、数字包括下划线:
^[\u4E00-\u9FA5A-Za-z0-9_]+$
- 中文、英文、数字但不包括下划线等符号:
^[\u4E00-\u9FA5A-Za-z0-9]+$ 或 ^[\u4E00-\u9FA5A-Za-z0-9]{2,20}$
- 可以输入含有^%&’,;=?$\”等字符:
[^%&',;=?$\x22]+
- 禁止输入含有\~的字符:
[^~\x22]+
附:正则表达式语法详解
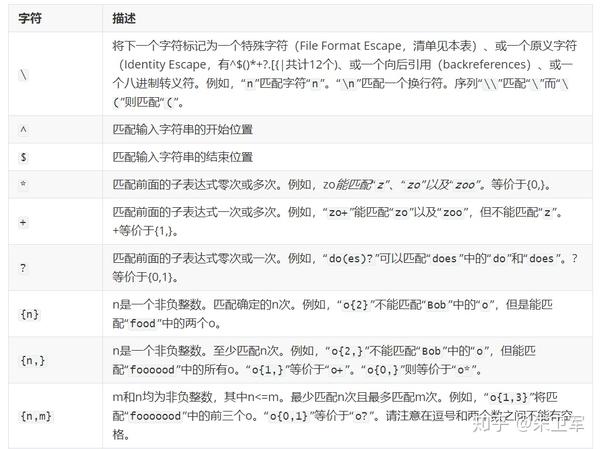
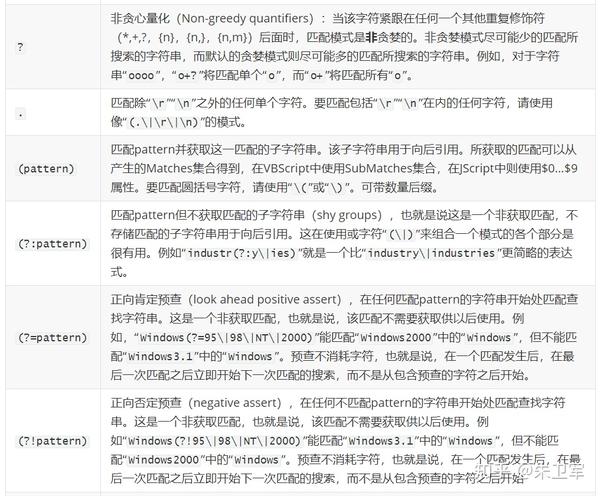
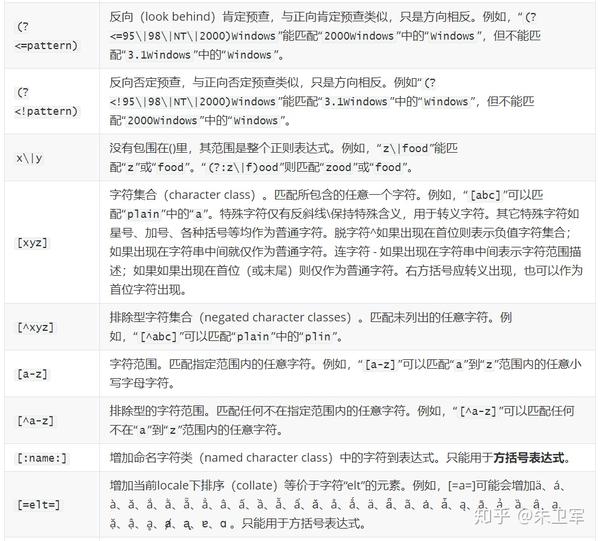
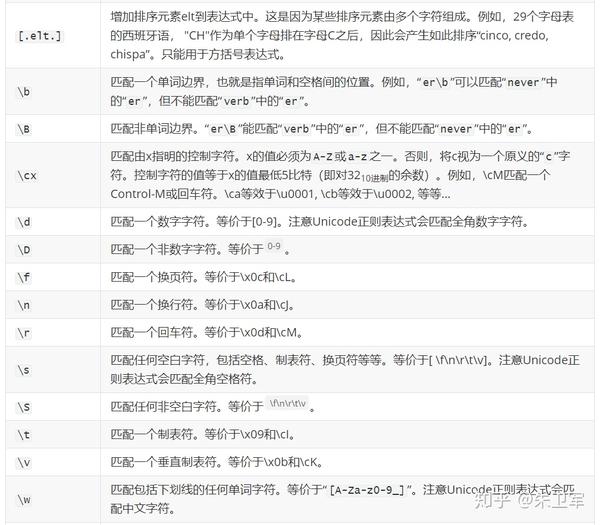
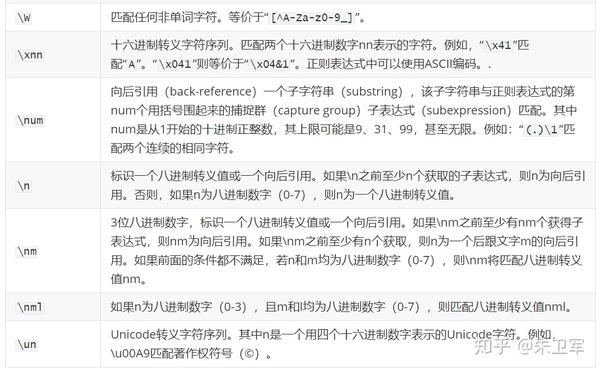
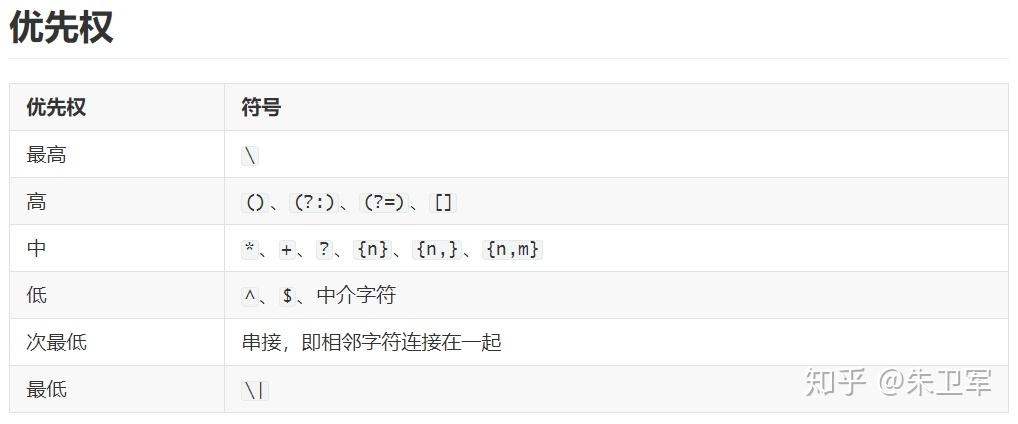