批量抢京东优惠券
By Vincent. @2020.6.1
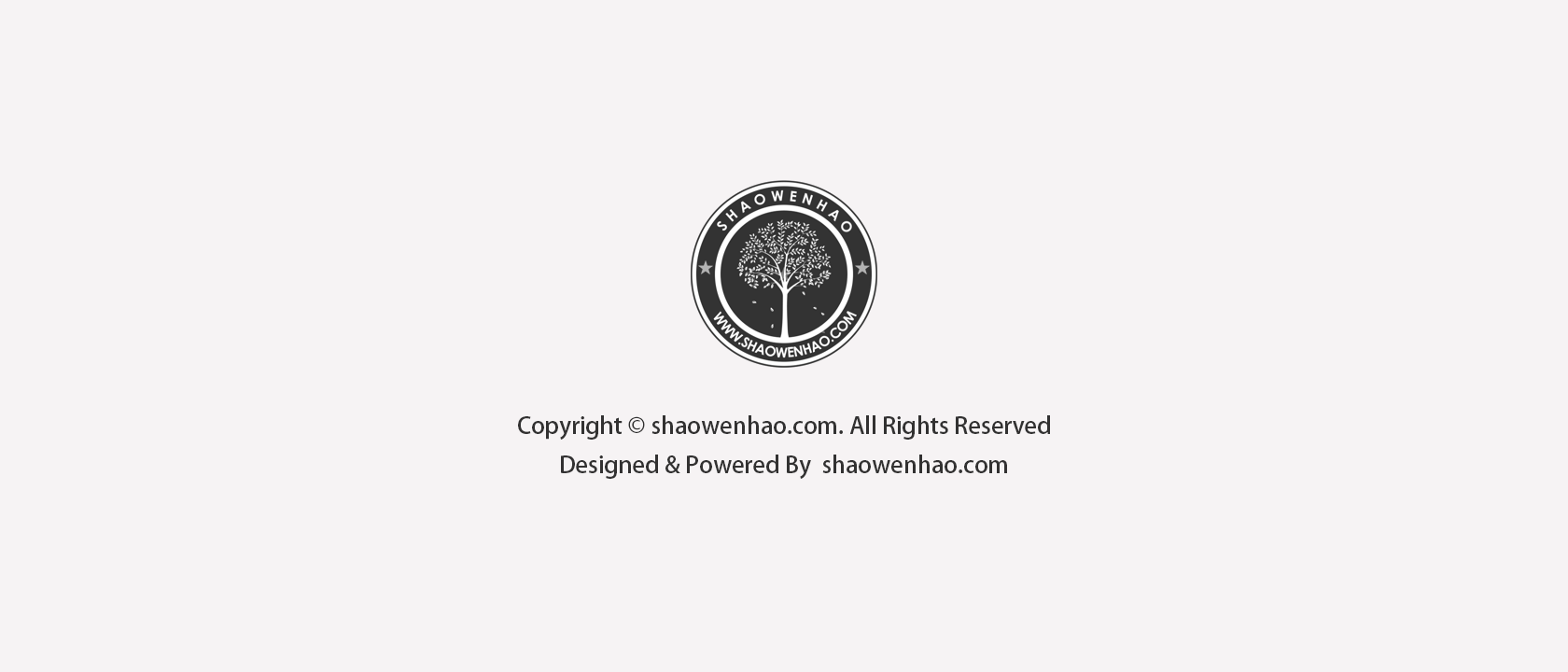
仅供学习交流,用于其他用途后果自负呦
你的cookies从哪来?
访问京东,登录账号后F12抓到log.gif的cookie全部内容复制过来即可
代码如下:
import requests
import json
import time
# 配置好自己的Cookies
my_cookies = '复制的Cookies'
timestamp = int(time.time() * 1000)
failedList = []
# 抢券并返回结果
def func_getCoupon(key, couponName, totalTime = 5):
global my_cookies
global timestamp
header = {'User-Agent': 'Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:64.0) Gecko/20100101 Firefox/64.0?', 'Referer': 'https://a.jd.com/', 'Cookie': my_cookies}
session = requests.session()
urlLogin = 'https://passport.jd.com/loginservice.aspx?&method=Login&_={0}'.format(timestamp)
response_login = requests.get(urlLogin, headers = header)
# https://a.jd.com/indexAjax/getCoupon.html?callback=jQuery6787354&key=0271DFD6890D3B60ACB8BA8A9E49BEB17FE8E6323A36834B63FE69E95D38088EB56E3FFE3F2EB2F5815B928F19275FC8D6B51563934644F28CBABCBD9E776F5353152AA954543CA6ACD9DB34AC3BB877CEFD4ED1C4A801F7FADF7E6B9A9D5EBB&type=1&_=1591065268687
url = 'https://a.jd.com/indexAjax/getCoupon.html?callback=jQuery6787354&key={key}&type=1&_={time}'.format(key = key, time = timestamp)
print('\n开始抢:' + couponName)
retryTimes = 0
while retryTimes <= totalTime:
response = requests.get(url, headers = header)
response.encoding = 'utf-8'
msg = json.loads(response.text[14:].replace(')', ''))
print('结果:' + msg['message'])
if '领券成功' in response.text:
return {'result': 1, 'couponName': couponName, 'msg': msg['message']}
elif '已经参加' in response.text or '只有专属用户可以领取' in response.text or '只有Plus正式期会员才能领取' in response.text or '仅家庭用户可以领取' in response.text or '仅店铺粉丝可以领取' in response.text:
return {'result': 2, 'couponName': couponName, 'msg': msg['message']}
elif '没有登录' in response.text:
return {'result': 3, 'couponName': couponName, 'msg': msg['message']}
else:
retryTimes += 1
if retryTimes <= totalTime:
print("还有希望,1秒后再次尝试( {now}/{total} )!".format(now = retryTimes, total = totalTime))
time.sleep(1)
if retryTimes > totalTime:
failedDic = {'couponKey': key, 'couponName': couponName, 'couponMsg': msg['message']}
failedList.append(failedDic)
print('疑似定时券!已暂存,下次运行会优先处理该优惠券')
return {'result': -1, 'couponName': couponName, 'msg': msg['message']}
# 按照分类 和 分类 逐一调用 func_getCoupon()抢券
def func_getCouponsList():
global my_cookies
global timestamp
succSum = 0;
header = {'User-Agent':'Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:64.0) Gecko/20100101 Firefox/64.0', 'Referer':'https://a.jd.com/'}
# https://a.jd.com/indexAjax/getCatalogList.html?callback=jQuery7901459&_=1591089496944 1591090222
url = 'https://a.jd.com/indexAjax/getCatalogList.html?callback=jQuery7901459&_={0}'.format(timestamp)
response = requests.get(url, headers = header)
catIDs = json.loads(response.text[14:].replace(')', ''))
for catID in catIDs['catalogList']: #分类
for pageID in range(1, 9): #分页
# https://a.jd.com/indexAjax/getCouponListByCatalogId.html?callback=jQuery4759758&catalogId=118&page=3&pageSize=9&_=1591064948304
urlListByCatalogId = 'https://a.jd.com/indexAjax/getCouponListByCatalogId.html?callback=jQuery4759758&catalogId={catid}&page={pageid}&pageSize=9&_={time}'.format(pageid = pageID, catid = catID['categoryId'], time = timestamp)
responseListByCatalogId = requests.get(urlListByCatalogId, headers = header)
items = json.loads(responseListByCatalogId.text[14:].replace(')', ''))
if items['totalNum'] == 0:
break
for item in items['couponList']:
couponName = '[{4}] {0}券 {1} ({2} {3})'.format(item['denomination'], item['quota'], item['limitStr'], item['discountDetailText'], catID['categoryName'])
iRet = func_getCoupon(item['ruleKey'], couponName)
if iRet['result'] == 1:
succSum += 1
print("本轮抢到 " + str(succSum) + " 张优惠券!\n")
return succSum
# 失败的优惠券 逐一调用 func_getCoupon()抢券
def func_getCouponByFailedList(failedList):
for failedCoupon in failedList:
# {'couponKey': key, 'couponName': couponName, 'couponMsg': msg['message']}
iRet = func_getCoupon(failedCoupon['couponKey'], failedCoupon['couponName'])
if iRet['result'] == 1:
failedList.remove(failedCoupon)
return failedList
# 读取暂存在配置文件中的优惠券信息
def func_readAndgetCoupon():
try:
with open('failedList.txt', 'r') as f:
failedList = f.read()# 一次性读全部成一个字符串
failedList = json.loads(failedList)
while len(failedList) > 0:
print('开始处理配置文件中的优惠券')
failedList = func_getCouponByFailedList(failedList)
try:
retryFlag = int(input('继续么?(1 继续)'))
except Exception as e_retryFlag:
break
if retryFlag != 1:
break
except Exception as e_func_readAndgetCoupon:
print('暂无配置文件,将进行遍历抢券')
# 主函数
def func_main():
global failedList
succSum = 0
# 抢本地暂存的异常券
func_readAndgetCoupon()
# 正常抢券
try:
times = int(input('抢几轮:'))
except Exception as e_times:
print("输入有误,将默认抢1论")
times = 1
try:
sleepTime = int(input('\n几秒抢1轮:'))
except Exception as e_sleepTime:
print("输入有误,将默认10秒抢1轮")
sleepTime = 10
print("抢券开始")
while times > 0:
try:
succSum += func_getCouponsList()
except Exception as e_func_getCouponsList:
succSum += 0
times -= 1
print (time.strftime("%Y-%m-%d %H:%M:%S", time.localtime()))
print(str(sleepTime) + " 秒后,将自动进行下一轮(还剩" + str(times) + "轮)...")
# 写入文件
with open('failedList.txt', 'w+') as fw:
fw.write(str(json.dumps(failedList, ensure_ascii = False)))
failedList.clear()
time.sleep(sleepTime)
print("抢券结束,共计抢到" + str(succSum) + "张")
# 执行主函数
func_main()